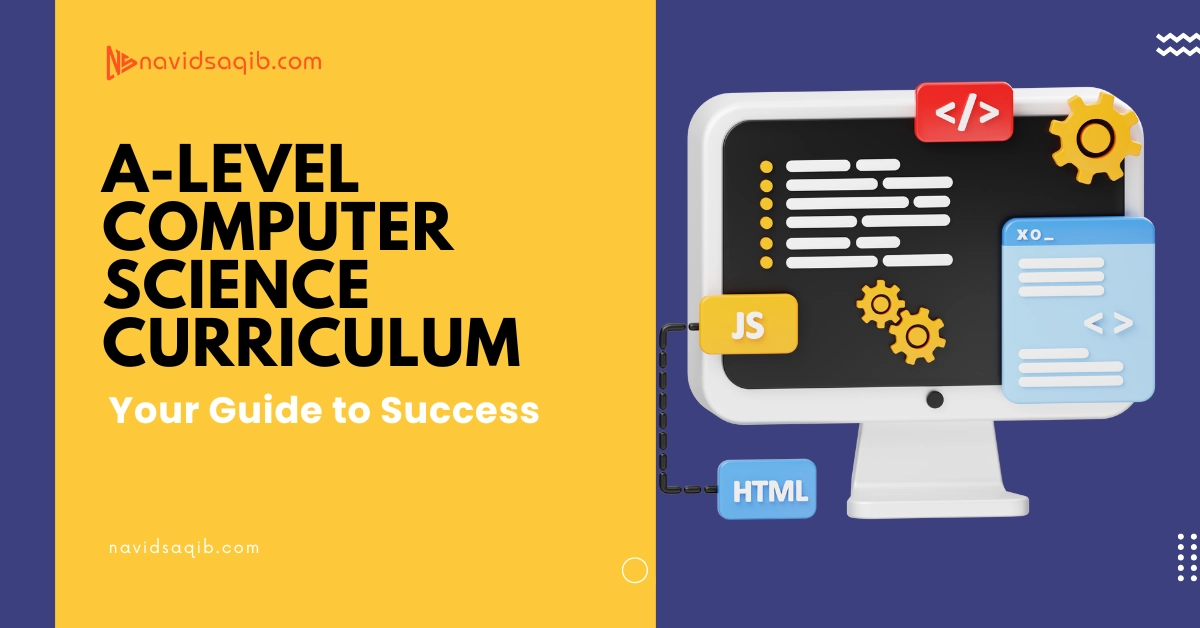
The goal of the comprehensive and in-depth A-Level Computer Science course is to give pupils a solid foundation in computing principles. A wide range of topics are covered in this course, from the fundamentals of programming to more intricate concepts in data structures, algorithms, and computer architecture.
It aims to increase students’ ability to think computationally, solve problems, and create and utilize effective software solutions.
Understanding computer science is more crucial than ever in the current digital era. It offers access to a wide range of employment opportunities in industries like technology, data science, artificial intelligence, cybersecurity, and software development.
Along with technical abilities, people who study computer science at the A-Level also acquire resilience, creativity, and logical thinking. These skills are not just helpful in tech-related organizations, but also in many other industries that rely on data and automation.
This guide will help you succeed in your A-Level Computer Science coursework by providing comprehensive explanations, practical examples, and useful advice. It will also open the door to further study or a future in the computer industry.
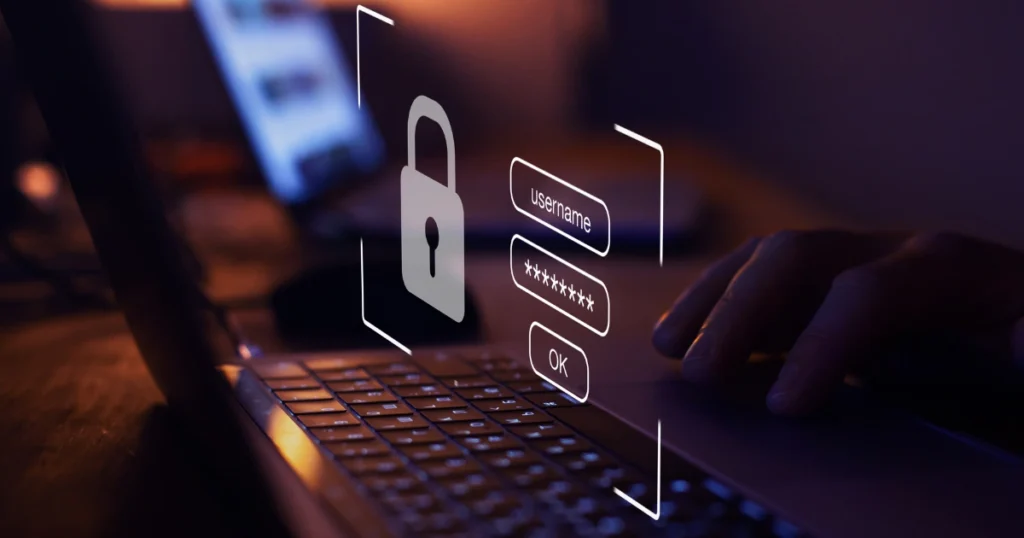
Stay Ahead in Your Studies!
Don’t miss out on valuable resources that can help you excel in your academic journey!
Overview of A-Level Computer Science Curriculum
The goal of the A-Level Computer Science curriculum is to give students a thorough understanding of the foundational ideas and concepts of computing. Students are prepared for both higher education and professions in technology by combining theoretical knowledge with practical abilities.
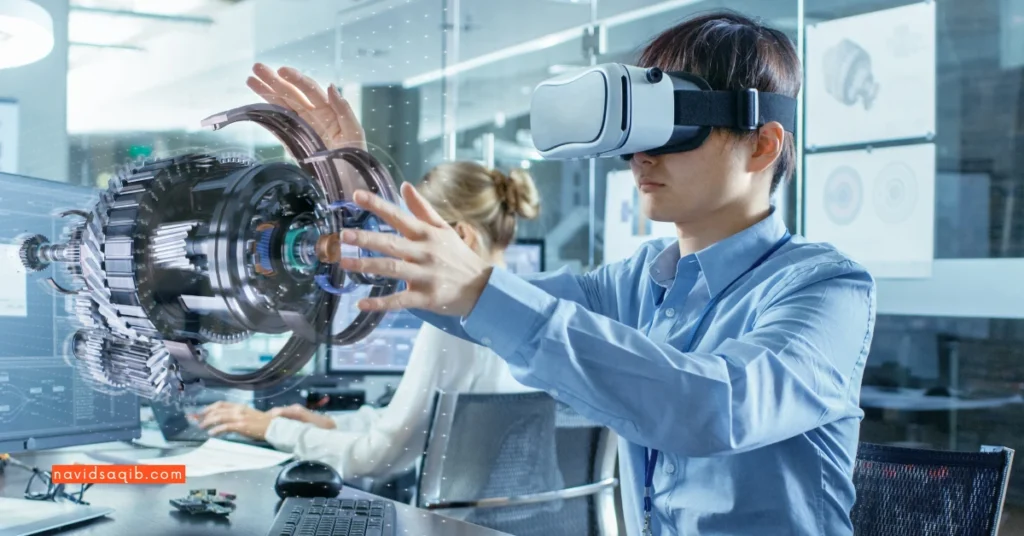
A balanced approach is ensured by the syllabus’s design, which covers both theoretical and practical aspects. Students gain knowledge of computer systems, networks, programming, algorithms, and data structures.
Students can apply their knowledge through coding projects in the practical components, while the theory sections highlight the science underlying how computers function.
This blend of theory and hands-on practice is crucial for developing problem-solving skills, logical thinking, and technical expertise.
Key Topics in A-Level Computer Science
Data Structures and Algorithms
Data structures and algorithms are fundamental to computer science, helping to organize and process data efficiently. Common data structures include:
- Arrays: Fixed-size structures for storing elements of the same type.
- Lists: Flexible, dynamic structures that can grow or shrink.
- Stacks: LIFO (Last In, First Out) structures used for tasks like undo functions.
- Trees: Hierarchical structures, including binary trees, which facilitate searching and sorting.
Students also learn various algorithms for data manipulation, including:
- Sorting Algorithms: Bubble sort, quick sort, and merge sort.
- Searching Algorithms: Linear search and binary search, which are used to locate data within structures.
Understanding these concepts is vital for writing efficient code and optimizing software performance.
Object-Oriented Programming (OOP)
Object-Oriented Programming is a programming paradigm that model’s software around real-world entities. Key concepts include:
- Classes and Objects: Classes are blueprints for creating objects, which represent specific instances.
- Inheritance: Allows new classes to adopt properties and behaviors from existing ones, promoting code reusability.
- Encapsulation: The practice of hiding internal states and requiring all interactions to occur through well-defined interfaces.
OOP is essential in modern software development because it supports modularity, scalability, and maintenance. Students learn to build robust applications using OOP languages like Python, Java, or C++.
Computer Systems and Architecture
Understanding computer systems and architecture is central to the A-Level syllabus. Students explore:
- CPU Operations: How the Central Processing Unit (CPU) processes instructions.
- Memory: Types of memory (RAM, ROM) and their roles in a system.
- Input/Output Devices: How peripherals like keyboards, printers, and monitors interact with the system.
These topics help students appreciate the inner workings of computers, from basic instruction execution to complex data processing.
Networking and the Internet
The networking section introduces students to the basics of how computers communicate. Key principles include:
- IP Addressing: Understanding how devices on a network are identified.
- Protocols: Rules that govern data exchange (e.g., TCP/IP, HTTP).
- Network Security: Techniques for securing data during transmission, including encryption and firewalls.
Students also learn about the significance of reliable and secure data transmission, which is crucial in today’s digital world.
A-Level Exam Format
Theory Paper Breakdown
Students’ understanding of basic computer concepts is assessed through the structure of the theory paper. It consists of essay-style questions that demand thorough justifications, multiple-choice questions, and short response questions.
Computer architecture, networking, algorithms, and data structures are important areas of study. It is required of students to exhibit a thorough comprehension of how these components function both separately and in combination.
Practical Paper and Coursework
During the practical component, students apply their theoretical knowledge through hands-on coding exercises. They create, implement, and test programs, which regularly tests their ability to produce understandable, practical code. The skills evaluated in this course include problem-solving, efficient code writing, and debugging and optimizing software solutions.
Exam Preparation Tips
Studying past papers, mastering theory and practical abilities, and comprehending the syllabus are all essential components of an effective A-Level Computer Science test preparation strategy.
Here are some strategies to consider:
- Time Management: Allocate specific times for studying theory, practicing coding, and reviewing past papers. This helps cover all areas systematically without last-minute cramming.
- Answering Theory Questions: Practice answering questions clearly and concisely. Focus on key points and avoid unnecessary details to manage time effectively during the exam.
- Solving Coding Problems: Regularly practice coding problems to build fluency in writing efficient code. Use online coding platforms to test your skills and familiarize yourself with different types of problems.
- Understanding Key Concepts: Focus on core topics like data structures, algorithms, and system architecture. Ensure you have a strong grasp of how each component works and interacts within the broader system.
- Mock Exams: Attempting mock exams under timed conditions can help identify areas where more practice is needed and improve overall exam performance.
Programming for A-Level Computer Science
One of the main components of the A-Level Computer Science curriculum is programming, which aims to give students the ability to write logical, well-structured, and efficient code.
Understanding and using different programming languages is emphasized in the curriculum, which teaches students how to use coding to address real-world problems.
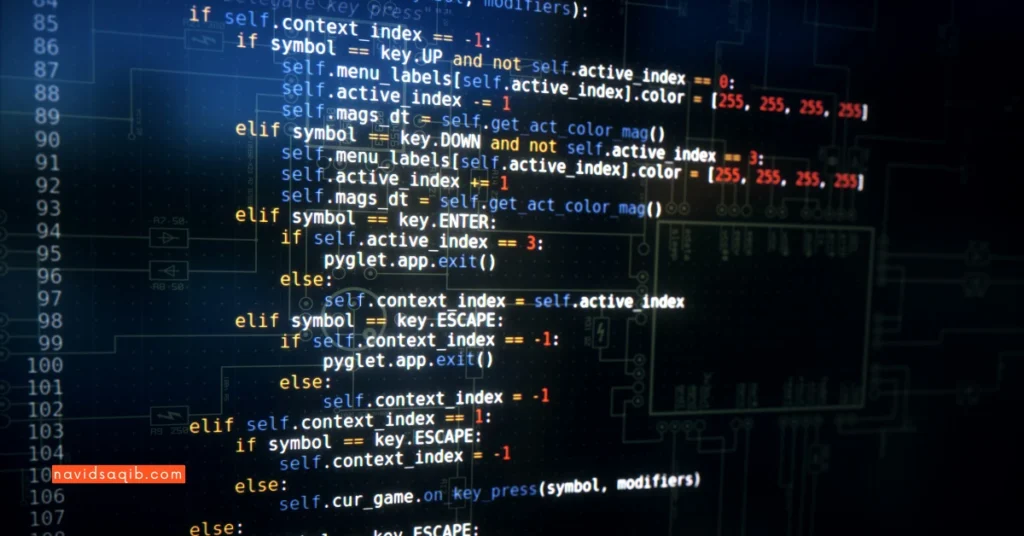
Students who learn to develop, debug, and optimize code get knowledge about the creation and upkeep of software applications.
A-Level programming courses also introduce ideas like data structures, algorithms, and computational thinking—all of which are critical for both higher education and jobs in data science, software development, and other tech-related industries.
Students can approach problems from different perspectives when they are proficient in many programming languages, which helps them get ready for increasingly challenging assignments in college or the workplace.
Recommended Programming Languages
Python
One of the most popular programming languages among students pursuing an A-Level is Python. Beginners can more easily and rapidly learn the fundamentals of programming because of its simple syntax. Python is incredibly flexible and can be applied to a wide range of tasks, including data analysis and web development.
Key concepts that A-Level students will learn include:
- Variables: How to store and manipulate data using variables.
- Loops: Understanding iteration through for and while loops.
- Functions: Creating reusable code blocks with parameters and return values.
- Error Handling: Techniques for managing exceptions and ensuring code runs smoothly.
Python’s simplicity and widespread use in the tech industry make it an excellent starting point for A-Level Computer Science students.
Java and C++
Java and C++ offer more complex programming principles that can be helpful for A-Level students hoping to study computer science at a higher level, even though Python is excellent for novices.
Learning these languages helps students understand the following:
- Data Structures: Mastery of arrays, linked lists, stacks, queues, and more.
- Object-Oriented Programming (OOP): Principles of encapsulation, inheritance, polymorphism, and abstraction.
- Memory Management: Directly managing memory allocation and deallocation in C++, which can be useful for developing a deeper understanding of how programs interact with hardware.
Students should consider learning Java or C++ if they plan to delve deeper into software engineering, game development, or systems programming.
Choosing the Right Language
The programming language selection must be in line with the particular specifications of the assignments or coursework.
For example, Java is better suited to creating scalable online applications, but Python is best suited for projects involving data. C++ might be the ideal option if the project—such as a software system or game needs performance optimization.
Before choosing which language to concentrate on, students should weigh the advantages and disadvantages of each, considering their professional objectives as well as the principles they want to learn about programming.
Practical Coding Projects
Project Ideas for A-Level
Engaging in practical projects helps students apply what they’ve learned and develop problem-solving skills.
Here are some project ideas for A-Level students:
- Simple Games: Create classic games like Tic-Tac-Toe, Snake, or Hangman, which introduce basic game logic and user input handling.
- Database Management Systems: Build a small-scale system that allows users to store, update, and retrieve data, helping students understand CRUD operations.
- Web Applications: Develop a basic website or web app using Python’s Flask or Django frameworks, incorporating backend functionality and user authentication.
These projects not only reinforce programming skills but also make for excellent additions to a student’s portfolio.
Best Platforms for Coding Practice
To build proficiency, students can use online platforms that offer a range of coding exercises, tutorials, and challenges.
Some recommended platforms are:
- HackerRank: Provides problems based on algorithms, data structures, and various programming languages, suitable for all skill levels.
- Codecademy: Offers interactive courses on multiple languages, with lessons designed to teach coding step-by-step.
- LeetCode: Primarily focused on algorithms and problem-solving, making it an excellent choice for students preparing for coding interviews or competitive programming.
Regular practice on these platforms can improve students’ coding abilities, helping them excel in their A-Level Computer Science exams and beyond.
Data Structures and Algorithms for A-Level
Effective programming and problem-solving require an understanding of fundamental computer science concepts including data structures and algorithms. A-Level students who understand these concepts are better prepared for further study and practical applications.
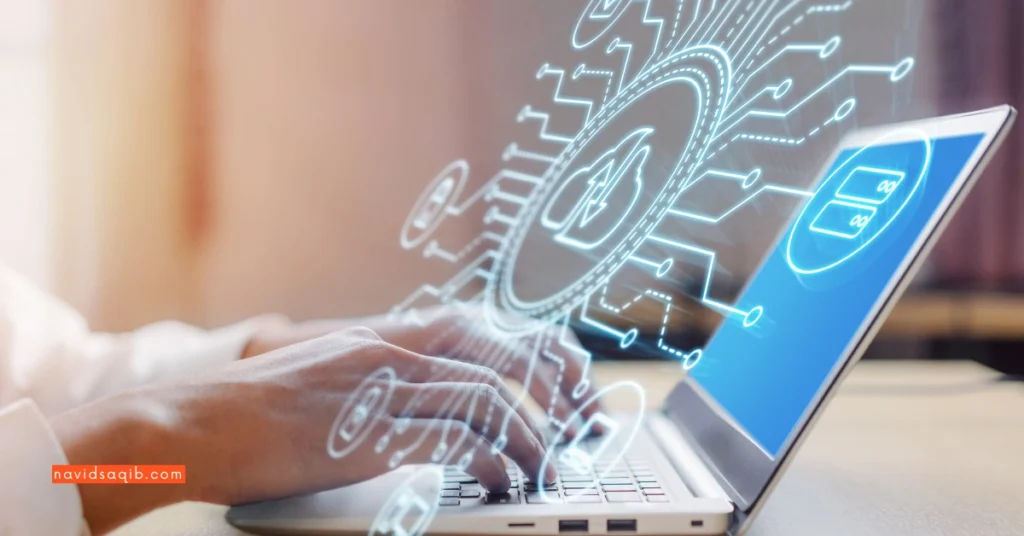
In contrast to data structures like arrays, linked lists, stacks, and queues, which efficiently organize data, algorithms provide comprehensive instructions for solving problems.
Understanding these will significantly improve your programming skills, allowing you to produce better software and perform well on A-Level exams.
Advanced Data Structures
Advanced data structures such as heaps, trees, and graphs play a crucial role in more complex problem-solving.
- Heaps: Efficiently manage priority queues and find the maximum or minimum element.
- Trees: Used in scenarios like file systems, databases, and expression parsing. Binary search trees, for instance, enable quick searching and sorting.
- Graphs: Represent networks of connections, such as social media platforms, roadmaps, and web structures. They help solve problems like finding the shortest path or optimizing routes.
These structures provide more versatile and efficient ways to handle and manipulate data compared to basic data structures.
Algorithm Design and Optimization
The goal of algorithm design is to provide accurate and effective solutions. Big-O notation, which illustrates how an algorithm’s performance varies with increasing input size, is frequently used to quantify efficiency.
Selecting or creating algorithms that operate more quickly and consume fewer resources is made easier by understanding time and space complexity.
Common algorithms include:
- Dijkstra’s Algorithm: Finds the shortest path between nodes in a graph, used in navigation systems.
- Dynamic Programming: Solves complex problems by breaking them down into simpler sub-problems, storing results to avoid redundant calculations.
- Recursion: Functions that call themselves to solve a problem, common in scenarios like tree traversal and factorial calculations.
These algorithms form the building blocks of more complex programming tasks, making them vital for A-Level students.
Coding Challenges for A-Level
To enhance algorithmic thinking, A-Level students should engage in coding challenges that test their understanding of data structures and algorithms.
Practice problems include:
- Array Manipulation: Rotating arrays, finding subarrays with specific properties.
- Tree Traversal: Pre-order, in-order, post-order traversal of binary trees.
- Graph Problems: Detecting cycles, finding shortest paths, and implementing breadth-first and depth-first search.
- Dynamic Programming: Solving problems like the Fibonacci sequence, knapsack problem, or matrix chain multiplication.
Consistent practice with these challenges will help students develop the skills necessary to tackle complex problems, making them better prepared for A-Level exams and future computer science studies.
Computer Systems and Hardware
A variety of hardware and software components work together to perform various tasks in computer systems. Hardware refers to the actual parts of a computer, including the hardware required for communication, data processing, and storage.
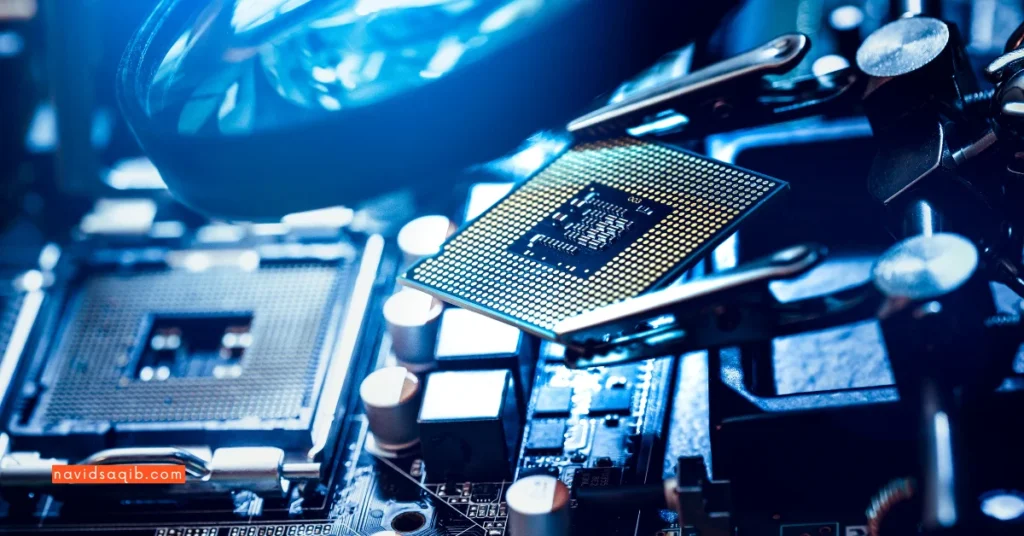
Both software, such as operating systems, and hardware components, such as CPUs, RAM, and I/O devices, are required for these systems to function properly.
Understanding the basic workings of operating systems and computer hardware is essential to comprehending how contemporary computers do jobs.
Components of a Computer System
A computer system consists of several key hardware components, each playing a crucial role in its functionality:
- Central Processing Unit (CPU): The CPU, sometimes known as the computer’s brain, does all mathematical and logical functions. It enables the system to perform tasks by processing and executing software instructions. Among the various components of the CPU are the control unit, which directs activities, and the Arithmetic Logic Unit (ALU), which does calculations.
- Random Access Memory (RAM): Short-term memory, or RAM, is where the system keeps information that the CPU needs to access quickly while working on tasks. Data in RAM is volatile, which means it is erased when the computer is shut down, in contrast to storage disks. The system’s capacity to manage several processes effectively is influenced by the amount of RAM.
- Input/Output (I/O) Devices: Computer-user interaction is made possible by peripherals known as I/O devices. Keyboards and computers are examples of input devices that allow users to enter data, and monitors and printers are examples of output devices that show or generate computer output. These gadgets help the computer and the outside world exchange data.
Operating Systems
The foundational software that controls a computer system’s hardware and software resources is called an operating system (OS). They provide a link between the machine’s hardware and its running applications and offer a user interface.
The OS handles multiple essential functions, such as:
- Process Management: Managing tasks and processes, ensuring efficient allocation of CPU time among various applications.
- Memory Management: Allocating memory resources to different processes and ensuring optimal performance. The OS manages the computer’s RAM and prevents memory conflicts.
- File System Management: Handling data storage, retrieval, and file organization on storage devices like hard drives and SSDs.
- Device Management: Coordinating input and output devices, ensuring the smooth operation of peripherals connected to the system. Popular operating systems include Windows, macOS, and Linux, each providing unique features and interfaces.
Memory Management
An essential function that guarantees the effective distribution, use, and release of memory resources in a computer system is memory management. It includes several methods that make computers function properly and avoid memory-related problems:
- Paging: The system uses paging, a memory management technique, to separate memory into pages, which are fixed-sized chunks. The system can execute processes without all data having to be loaded into physical memory thanks to these pages that are mapped between physical and virtual memory. Paging makes greater use of the available RAM and lessens memory fragmentation.
- Segmentation: Segmentation separates memory into variable-sized chunks according to logical divisions inside a program, like code, data, or stacks, in contrast to paging. The system has greater flexibility in accessing various program components since each segment is handled as an individual. Memory use is improved by segmentation, however improper management might result in external fragmentation. The stability and performance of the computer are maintained by efficient memory management, which guarantees that programs may operate without using excessive amounts of resources.
Advanced Computer Architecture
Advanced computer architecture explores the intricate structure of computer systems, highlighting the ways in which many components work together to maximize performance and efficiency.
This includes CPU architecture and operation, networking concepts that let computers connect to other networks, and low-level programming using assembly language.
It is essential to comprehend sophisticated architecture concepts if one wants to build, optimize, or troubleshoot modern computer systems.
CPU Design and Functionality
The Central Processing Unit (CPU) is the core of any computer system, responsible for executing instructions. It consists of three primary components:
- Arithmetic Logic Unit (ALU): The ALU performs all arithmetic and logical operations, including addition, subtraction, comparisons, and logical operations.
- Control Unit (CU): The CU manages the execution of instructions by directing the flow of data within the CPU. It interprets the instructions fetched from memory and coordinates the activities of other components.
- Registers: The CPU has tiny, fast-moving storage spaces called registers. Compared to main memory, they provide for faster access by temporarily storing data during processing. Registers serve as the workspace for the CPU and are used for fast computations and control commands. Together, these parts process commands from software programs, carry out computations, and regulate the computer’s data flow.
Assembly Language
A low-level programming language called assembly language offers a means of writing instructions that the CPU can understand. Assembly language is closely tied to the machine code that the CPU runs, in contrast to high-level languages like Python or Java that are abstracted and simpler for humans to read.
Instructions are represented by mnemonic codes, such as ADD for addition or MOV for moving data.
When creating software that necessitates direct connection with the system’s components, assembly language writing gives programmers precise control over the hardware. High-level languages may introduce inefficiencies in performance-critical operations, which it also helps to optimize.
Networking Fundamentals
Networking fundamentals encompass the principles that enable computers to communicate and share data across different systems.
Key concepts include:
- TCP/IP Protocol: Communication over the internet is based on the Transmission Control Protocol/Internet Protocol (TCP/IP). TCP establishes connections and checks for errors to ensure dependable data delivery, whereas IP manages packet routing between sender and recipient.
- Packet Switching: Data is divided into smaller packets and delivered separately via the network, where they are reassembled at the destination. This process is known as packet switching. This technique minimizes congestion and ensures dependable communication while enabling the effective use of network resources.
- Protocols: The guidelines that control data transmission between devices are known as protocols. SMTP is used for sending emails, FTP is used for file transfers, and HTTP is used for online browsing. Every protocol specifies the format, transmission, and reception of data. For smooth data transfer between devices, network connection setup, management, and troubleshooting require a solid understanding of networking principles.
Practical Coursework and A-Level Projects
Students can apply theoretical knowledge in real-world situations and learn via experience with A-Level programming practical training. Students gain valuable skills like technical proficiency, project management, and problem-solving through projects.
A student’s ability to plan, execute, and debug a complete software solution is another ability demonstrated by these tasks, which is highly regarded for future coursework or tech-related jobs.
Overview of the Programming Project
Students working on A-Level programming assignments usually have to develop a workable software program that addresses a particular issue. Issue identification, system design, coding, testing, and documentation are some of the steps in the project process.
Students must adhere to established procedures in order to guarantee the efficient completion of each phase. Simple calculators to more intricate systems like inventory management software are examples of projects.
The principal aim is to demonstrate a comprehensive comprehension of programming ideas, logical reasoning, and best practices for coding. Because it aids in illustrating the technical procedures and thought process used to produce the finished product, proper documentation is also essential.
How to Approach the A-Level Project
Choosing the appropriate project topic is essential to its success. Select a subject that allows for the demonstration of a range of abilities while remaining both difficult and doable. Begin by generating ideas and looking for related projects to get ideas.
The next step is planning; establish a project schedule with due dates and milestones for every stage. Starting with the fundamental framework and gradually adding features should be the approachable first step in implementation. To find problems early, testing and debugging are essential.
Lastly, record the entire procedure, including any difficulties encountered and their solutions. This improves problem-solving skills in addition to project evaluation.
Best Tools for A-Level Projects
Using the appropriate tools can make project development much easier. For coding, Integrated Development Environments (IDEs) such as Visual Studio Code, PyCharm, or IntelliJ IDEA are great since they include debugging tools, code suggestions, and syntax highlighting.
Error troubleshooting requires the use of debugging tools; programs such as GDB for C++ or PDB for Python can effectively find and address flaws. For managing code versions, monitoring changes, and working with peers, version control systems like Git are also strongly advised.
Projects can be stored and shared via GitHub and GitLab, which facilitates collaboration with team members or across devices.
Top Project Ideas
Selecting a project topic that reflects your hobbies will increase the level of engagement and enjoyment during the creation process.
Here are some suggestions that span from simple to complex programming fields.
Database Management Systems
Building a basic database management system (DBMS) is a great method to learn about data manipulation, retrieval, and storage. For relational databases like MySQL or PostgreSQL, use SQL; for unstructured data, try NoSQL databases like MongoDB for greater flexibility.
Students can create a database management system (DBMS) that keeps student records, maintains inventory, or even indexes library books. This project will instruct students in topics such as data normalization, query optimization, and database design.
Web Application Development
For students interested in front-end and back-end development, creating a tiny web application is an excellent assignment. For the front end, you can start with simple technologies like HTML, CSS, and JavaScript. For the back end, you can use Python (with Flask or Django), PHP, or Node.js.
Potential tasks include developing a simple e-commerce platform, an online to-do list, or a personal portfolio website. You will get knowledge about client-server architecture, responsive design, and web development frameworks from this project.
Machine Learning Projects
Machine learning projects are a demanding yet worthwhile option for advanced students wishing to delve deeper into artificial intelligence. You may create easy applications like a spam email classifier, sentiment analysis tool, or even a simple picture recognition system with Python frameworks like TensorFlow, scikit-learn, or Keras.
These projects offer practical expertise with algorithms, prepping data, and training models, as well as insights into the rapidly expanding fields of artificial intelligence and machine learning.
A-Level Computer Science Resources
When preparing for A-Level Computer Science, a strong foundation in theoretical principles, problem-solving techniques, and coding must be established through the use of a range of excellent resources.
Students can use a variety of resources, such as textbooks, internet resources, and prior papers, to better understand the material and perform well on exams.
The best resources for enhancing your A-Level computer science studies are examined below.
Best Textbooks for A-Level
Selecting the right textbooks is crucial for mastering A-Level Computer Science. Here are some highly recommended books:
- “Cambridge International AS and A Level Computer Science Coursebook” by Sylvia Langfield and Dave Duddell – A comprehensive guide that covers both the AS and A-Level syllabus. It offers clear explanations, worked examples, and exercises.
- “AQA A Level Computer Science” by Bob Reeves – Tailored specifically for the AQA exam board, this textbook breaks down complex topics into easy-to-understand sections, with plenty of practice questions.
- “OCR AS and A Level Computer Science” by P. M. Heathcote and R. S. U. Heathcote – Ideal for students following the OCR curriculum, this book covers theory and programming concepts with step-by-step explanations and code examples.
These textbooks are designed to help students understand fundamental concepts, programming techniques, and advanced topics covered in the A-Level syllabus.
Online Resources for Learning
The internet is a treasure trove of resources for A-Level Computer Science students. Some of the best online platforms and courses include:
- Coding Platforms: Websites like Codecademy and LeetCode provide hands-on coding practice that can improve your programming skills.
- Online Courses: Platforms such as Coursera, Udemy, and edX offer courses on topics ranging from programming basics to advanced computer science concepts. Popular courses include “Python for Everybody” on Coursera and “Introduction to Algorithms” on edX.
- Video Tutorials: YouTube channels like The Coding Train, Derek Banas, and Programming with Mosh offer tutorials that explain coding concepts in a simple and engaging way, making it easier to learn at your own pace.
These online resources help students reinforce their understanding of complex topics and offer additional practice beyond textbooks.
Past Papers and Marking Schemes
Practicing with past papers is one of the most effective ways to prepare for A-Level Computer Science exams. Here’s why:
- Familiarity with Exam Format: Past papers allow students to get acquainted with the structure and style of questions that are typically asked in exams.
- Time Management: Solving past papers under timed conditions helps students improve their speed and accuracy, which is essential for achieving high scores.
- Understanding Mark Schemes: Reviewing marking schemes alongside past papers can help students understand how marks are awarded. This enables them to focus on key points that are essential for gaining full marks in answers.
Students can find past papers and marking schemes on exam board websites (like Cambridge International, AQA, and OCR) or educational platforms that offer a wide range of A-Level resources.
Conclusion
In this tutorial, we examined important concepts and strategies to help you succeed in A-Level Computer Science. Important topics covered included programming basics, data structures, algorithms, and the need of understanding computational thinking.
We also talked about effective study techniques including consulting earlier papers, taking part in group discussions, and using online guides and tools.
To succeed in A-Level Computer Science, students must stick to a study schedule, practice coding often, and make use of the wealth of resources available, including textbooks, coding platforms, and online courses.
Engaging with the information and applying what you have learned through practical exercises will help you build a stronger foundation and enhance your problem-solving skills. You will be successful in your A-Level Computer Science studies if you continue to be curious and hardworking!
FAQs
1: What programming language should I learn for A-Level Computer Science?
Learning Python is helpful for A-Level Computer Science Curriculum because of its simplicity and readability, which make it popular in the classroom. Java is also well-liked for teaching ideas related to object-oriented programming. You will have a strong foundation in programming concepts and problem-solving abilities if you get familiar with these languages.
2: What are the most important topics in A-Level Computer Science?
Key topics typically include:
- Programming concepts: Understanding algorithms, data structures, and programming languages.
- Computational thinking: Problem-solving techniques and algorithm design.
- Data representation: How data is stored and manipulated in computers.
- Computer systems: Understanding hardware components, operating systems, and networks.
- Software development: Principles of software design, testing, and documentation.
- Ethical, legal, and environmental impacts of computing: Issues surrounding technology use in society.
3: How can I prepare effectively for the A-Level Computer Science exam?
To prepare effectively:
- Review the syllabus: Understand the topics covered in your syllabus.
- Practice past papers: Regularly solve past exam papers to familiarize yourself with the question format and timing.
- Join study groups: Collaborate with peers for discussions and problem-solving.
- Utilize online resources: Platforms like Codecademy and Coursera offer courses that can supplement your learning.
- Focus on weak areas: Identify topics you find challenging and dedicate time to improve in those areas.
4: Where can I find past A-Level Computer Science papers?
The official exam board websites (such AQA, Edexcel, or OCR), study material platforms, or educational resource websites like Revision World are all good places to find previous A-Level Computer Science problems. Past papers are also accessible through the web portals of several schools.
5: How should I approach my A-Level Computer Science project?
When approaching your A-Level Computer Science project:
- Choose a relevant topic: Select a project that interests you and aligns with your skills.
- Plan thoroughly: Outline your project goals, timeline, and required resources.
- Research extensively: Understand the theoretical concepts and existing solutions related to your project.
- Implement in stages: Break the project down into manageable sections, coding and testing each part before moving on.
- Document your progress: Keep track of your development process and challenges faced for your final report.
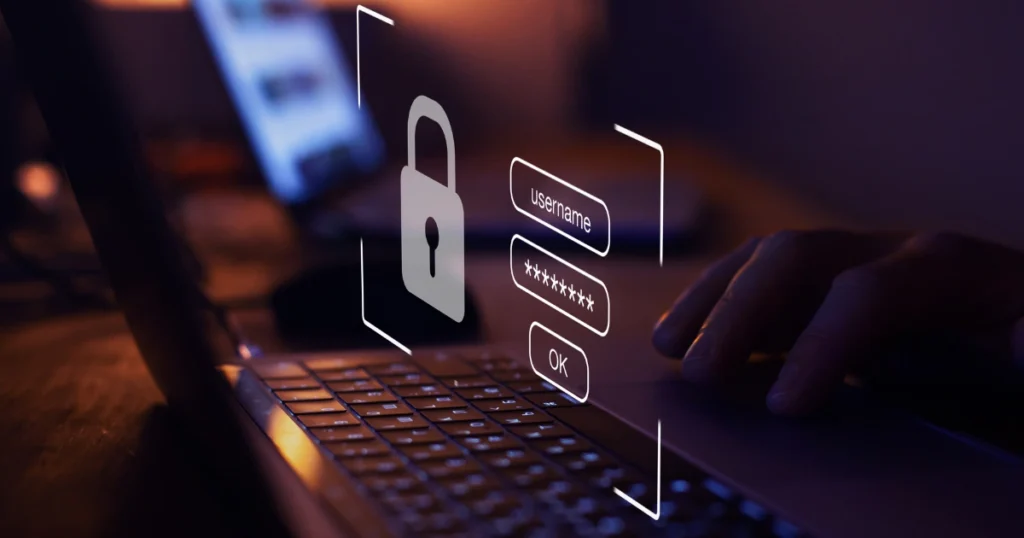
Stay Ahead in Your Studies!
Don’t miss out on valuable resources that can help you excel in your academic journey!