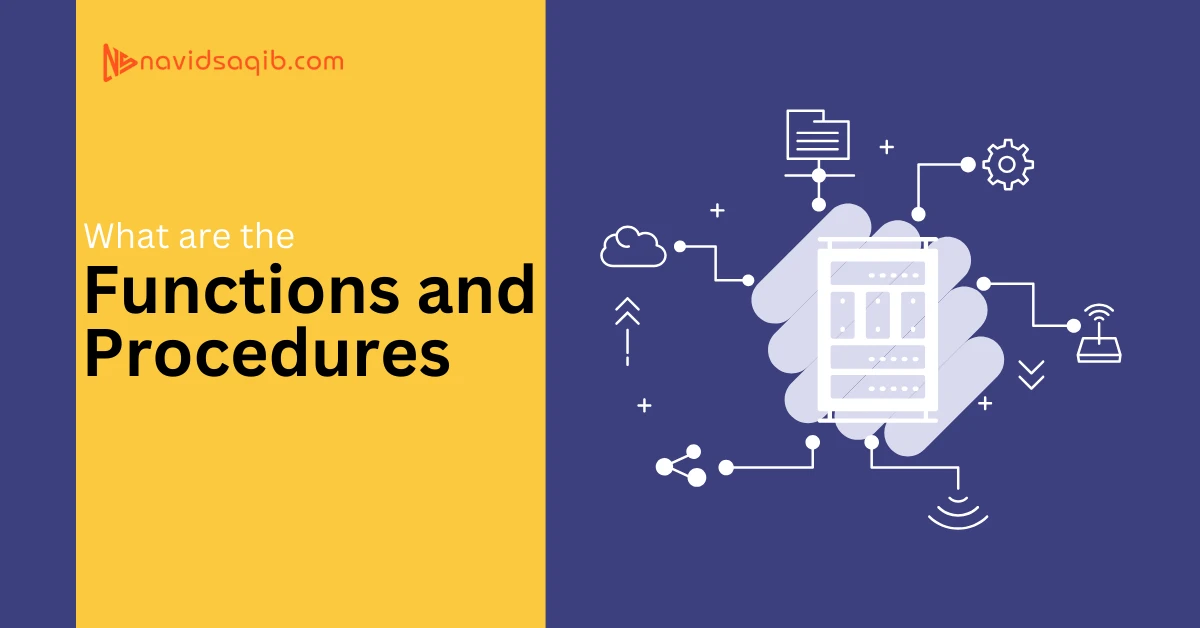
In the world of programming, organizing code in a structured and reusable manner is key to writing efficient, maintainable, and scalable software. Two fundamental concepts that help achieve this are functions and procedures.
While both serve as blocks of code that perform specific tasks, they differ significantly in their purpose, behavior, and how they are used within a program.
A function is designed to perform a particular task and return a value, enabling it to be used in expressions or as part of a larger operation. On the other hand, a procedure focuses on carrying out an action or operation without returning any result, often used for its side effects like modifying data or outputting information.
Understanding the distinction between these two concepts is crucial for any programmer, as it influences how code is structured, how operations are carried out, and how results are processed within a program.
In this article, we will explore the definitions, characteristics, and key differences between functions and procedures, helping you understand when and how to use each in your coding projects.
Key points of comparison
We will delve into the definitions and characteristics of both functions and procedures, comparing them in key areas such as:
- Return Values: Functions typically return a value, while procedures do not.
- Purpose and Usage: Functions are used to compute results that can be used in expressions, while procedures execute actions that might modify the program state but do not produce a return value.
- Syntax and Structure: How functions and procedures are written and the differences in their implementation.
- When to Use Each: The practical applications of both, and when to choose one over the other depending on the programming scenario.
By the end of this article, you will have a clear understanding of how and when to use functions and procedures effectively in your programming projects.
Function in Programming
A function is a block of programming code designed to perform a specific task and return a value. Functions are fundamental to most programming languages and are essential for writing efficient and reusable code.
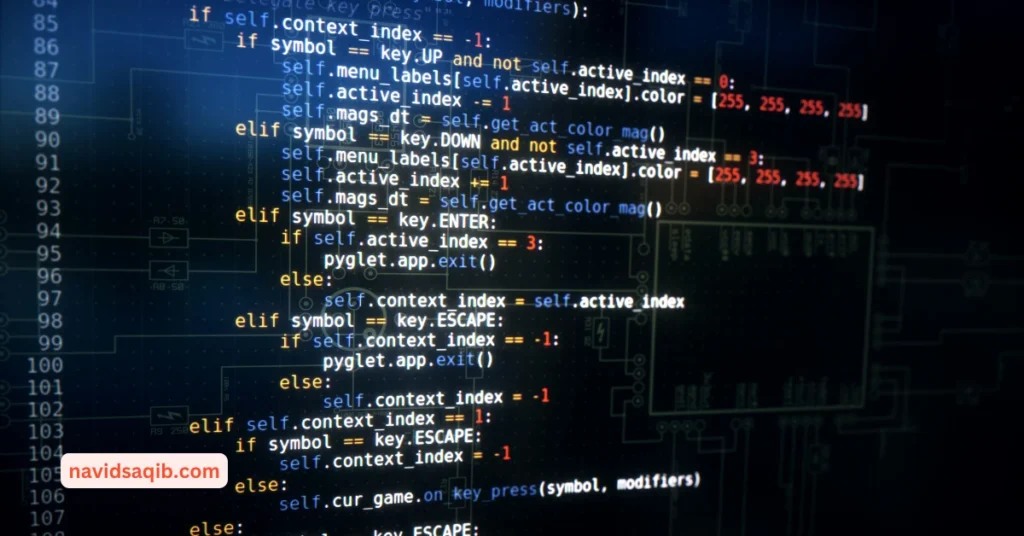
They help in breaking down complex problems into smaller, manageable parts, and they enable you to perform operations with specific inputs to produce a desired output.
Characteristics of a Function:
The characteristics of a Function are explained below:
Return Value: One of the defining characteristics of a function is that it returns a value. This return value could be any data type, such as integers, strings, arrays, or more complex data structures. The value returned by a function can be used elsewhere in the program.
Input Parameters (Arguments): Functions can take one or more input parameters, also known as arguments. These inputs allow the function to process specific values passed to it during execution. The arguments can vary in type and number depending on the function’s design.
Reusability: Functions promote code reusability. Once a function is defined, it can be called multiple times from different places in the program, saving time and reducing the need to duplicate code.
Encapsulation: Functions encapsulate specific behavior or logic. By using functions, you can hide the complexity of a task and simply call the function to perform a task without worrying about its internal workings.
Modularity: Functions help in organizing code into smaller, self-contained modules. This makes the program easier to read, maintain, and debug.
When to Use a Function:
Functions are ideal when you need to perform a specific operation or calculation multiple times within your program.
- Repetitive Tasks:
When a specific task or operation needs to be performed multiple times throughout your program, a function allows you to define the task once and reuse it without duplication. This reduces code repetition, making the program easier to maintain and modify.
Functions are a key part of structured programming. They allow for cleaner, more modular code that is easier to maintain and understand.
- Data Transformation or Calculation:
Functions are commonly used to perform mathematical or logical operations that take inputs, process them, and return a result. This includes operations such as calculations, data filtering, sorting, or aggregating values.
- Simplifying Complex Code:
If your program contains a complex set of operations or algorithms, breaking the code into smaller functions can make it more understandable and easier to debug. Functions allow you to encapsulate complex logic within a single block of code, making the main part of your program cleaner and more readable.
- Modularity and Reusability:
Functions allow for modularity in programming. A well-defined function performs one specific task, and as a result, it can be reused across different programs or projects. This modular approach helps with the organization and scalability of the code, especially in larger software systems.
- Handling Repetitive Input-Output Operations:
Functions are ideal when you need to repeatedly process or validate input from users or interact with external systems, like databases, APIs, or files. By isolating these tasks into functions, you ensure that your program can handle various inputs in a consistent and controlled manner.
You should use functions when:
- You need to perform a task multiple times.
- You need to process input and return output.
- You want to break down complex logic into simpler, reusable parts.
- You want to improve code maintainability, readability, and modularity.
- You are working with large programs and want to organize your code effectively.
Functions allow for better management of your codebase and play a critical role in building efficient, clean, and well-structured programs.
How functions are used to return a value?
Functions in programming are designed to perform specific tasks and return values. The concept of returning a value from a function allows you to pass results back to the caller, enabling further operations based on that result. The return statement is the mechanism by which a function outputs a value to the calling code.
When a function is called, it processes the input (if any), performs its task, and then sends a result back to the calling environment. This result is typically the “output” of the function, which can then be used in further calculations, stored in variables, or passed to other functions.
Key Points of Returning Values from Functions:
- The Return Mechanism:
Functions use the return statement to send a result back to the caller. Once the function reaches the return statement, it stops executing and sends the specified value back. - Return Value Types:
Functions can return various types of data, including numbers, strings, lists, or even complex objects. This versatility allows functions to be used for a wide range of tasks, from basic arithmetic to more complex data manipulation. - Using Return Values:
The value returned by a function can be used directly in expressions, stored in variables, or passed as input to other functions. This ability to return values enables functions to act as building blocks in larger programming tasks. - Multiple Return Values:
Some functions are capable of returning multiple values, often bundled together as a collection, like a list or tuple. This allows functions to return more than one piece of information simultaneously. - No Return Value (None):
In certain cases, a function may not return any value. In such situations, the function implicitly returns None (in some programming languages). This is typical for functions that perform actions, such as printing to the screen or modifying global variables but don’t need to provide a result.
Functions are used to return values that can be utilized elsewhere in the program. This feature enhances modularity and reusability by allowing parts of a program to interact and pass data efficiently.
Examples of Function Usage in Different Programming Languages
Functions are used across various programming languages, and although the syntax might differ, the core concept remains the same: to perform a task and return a result. Here are some examples of how functions are used in popular programming languages like C++, Python, and others.
1. C++ Example:
In C++, a function is defined using the return type, function name, and optional parameters. The function can then return a value using the return statement.
- Function Definition: A C++ function typically includes a return type, such as int, float, void (for functions that don’t return a value), and the name of the function followed by parameters.
Example: A function to calculate the sum of two numbers:
In C++, a function can be used to calculate the sum of two numbers and return the result.
- Code Overview:
- A function sum() is defined to take two integers as arguments and return their sum.
- The main() function calls sum() and stores the result in a variable.
- The result is then printed to the console.
2. Python Example:
Python has a simpler syntax for defining functions compared to C++, but it follows the same basic principles: define the function, call it, and return a value.
Example: A function to calculate the area of a circle:
In Python, a function can calculate the area of a circle based on a given radius and return the result.
- Code Overview:
- The function area_of_circle() takes a parameter radius.
- It computes the area using the formula π * r^2 and returns the result.
- The returned value is stored in the variable area, and the result is printed.
3. JavaScript Example:
In JavaScript, functions can be defined using the function keyword (or using arrow functions in modern JavaScript), and they can return values just like in C++ and Python.
Example: A function to multiply two numbers:
In JavaScript, a function can take two numbers, multiply them, and return the result.
- Code Overview:
- The function multiply() takes two numbers as arguments.
- It multiplies them and returns the result.
- The result is then displayed on the console.
4. Java Example:
In Java, functions are called methods and are always defined within a class. The concept of returning values works similarly to C++ and Python.
Example: A function to check if a number is even:
In Java, you can define a method to check whether a given integer is even, returning true if it is and false otherwise.
- Code Overview:
- The isEven() method takes an integer as a parameter and checks if it is divisible by 2.
- The result (either true or false) is returned.
- The main method calls isEven() and prints the result.
Procedure in Programming
A procedure is a block of code in programming that performs a specific task or action but does not return a value to the caller. Procedures are often used to execute operations, modify program state, or perform actions like displaying information or modifying data structures, without needing to produce a return value.
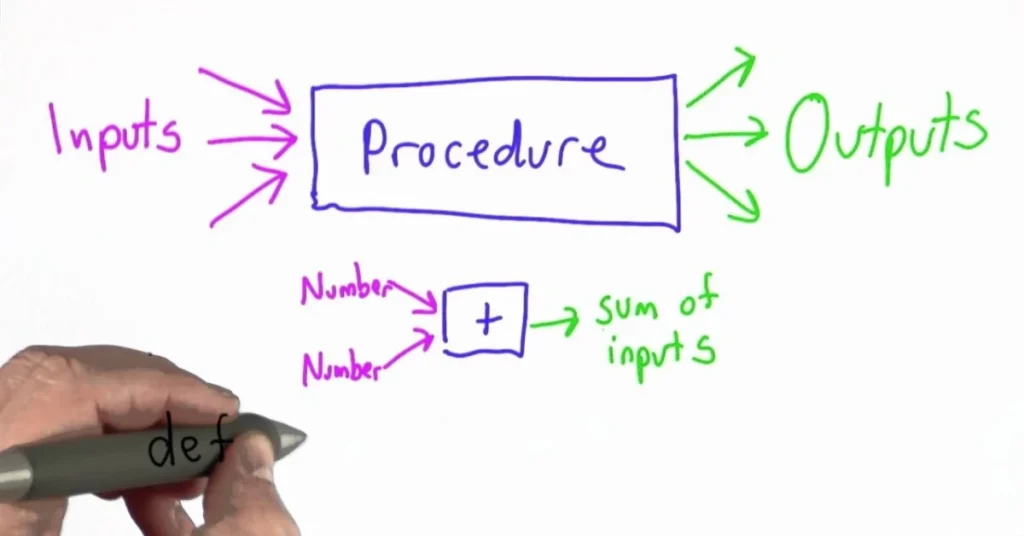
While a function typically returns a value, a procedure’s primary purpose is to execute code that has side effects, such as printing output or modifying variables.
Characteristics of a Procedure:
- No Return Value:
Unlike functions, procedures do not return any value. Instead, their primary purpose is to carry out an operation or sequence of instructions, often modifying variables or performing actions that affect the program state, such as interacting with the user or updating data. - Action-Oriented:
Procedures are action-driven, meaning they are focused on performing tasks. These tasks could include things like updating a database, sending data to an external system, or performing a series of operations that don’t need to be returned. - Parameters (Optional):
Like functions, procedures can accept parameters or arguments, allowing them to work with external data. However, while functions often use their parameters to produce a result, procedures may use their parameters to modify state or perform actions based on them. - No Return Statement:
Procedures typically do not include a return statement. While a function will use return to pass back a result, a procedure may simply execute commands without returning a value to the calling code. - Side Effects:
The operations performed by procedures usually lead to side effects, which are changes to the program’s state. For example, a procedure might change the value of a variable, print a message to the screen, or modify the contents of a data structure. - Reusability:
Just like functions, procedures can be reused throughout the program. By isolating a task into a procedure, the code can be cleaner and more modular, as the procedure can be called multiple times wherever that specific action is needed. - Example of Procedure Use:
A procedure could be used to log an error message to a file, update a user interface, or perform a complex series of operations that doesn’t require a return value, such as initializing settings.
When to Use a Procedure
Procedures are primarily used when a task or action needs to be performed without the requirement of returning a value. They are suited for scenarios where the focus is on executing operations or affecting the program’s state rather than producing a result to be passed back to other parts of the program.
Here are several key situations when using a procedure is beneficial:
- Performing Tasks Without Returning a Result:
Use a procedure when the task doesn’t require a return value. For example, if you need to display a message on the screen, log information to a file, or update a user interface, a procedure is appropriate since these actions don’t need to return any data to the caller.
- Modifying Program State or Variables:
Procedures are ideal for tasks that involve modifying the state of variables or the overall program environment. For instance, changing the value of a global variable, updating a record in a database, or modifying an object’s state should be done using procedures.
- Performing Operations with Side Effects:
Side effects are changes that occur outside the function or procedure’s immediate environment, such as writing data to a file, changing the contents of a data structure, or interacting with external systems. Procedures are typically used to handle side effects, where you need to act without worrying about returning a result.
- Encapsulating Repeated Tasks:
When you need to perform a task repeatedly throughout the program, but the result of that task doesn’t need to be returned, you can define a procedure to avoid duplicating the code. For example, procedures can be used for tasks like resetting settings, printing headers, or logging events.
- Interacting with External Resources:
Procedures are commonly used to interact with external resources such as databases, APIs, or hardware. For example, when sending data to an API, processing a file, or updating a record in a database, you usually don’t need a return value; instead, you just want to complete the action.
Use a procedure when the task involves performing actions such as modifying program state, printing outputs, or interacting with external systems, without the need for a return value.
How Procedures Differ from Functions: Specifically in Terms of Not Returning a Value
While both procedures and functions are subprograms designed to perform specific tasks, their key distinction lies in the concept of returning a value.
- Return Value:
Functions: A function always returns a value. The purpose of a function is typically to perform a task and return a result that can be used or stored by the calling code. For example, a function may compute the sum of two numbers and return the result to the caller.
Procedures: A procedure does not return a value. Instead, its purpose is to execute a set of instructions or perform actions, often affecting the program’s state (e.g., modifying a variable or displaying output), without needing to provide a result to the calling code.
- The focus of Use:
Functions: Functions are used when you need to calculate or produce a result that will be used later in the program. They perform a computation or logic and send a value back to the caller.
Procedures: Procedures are used when you need to perform an action or carry out a task without expecting a value to be returned. These actions may include operations like logging information, printing messages, updating a database, or altering the state of variables, but they don’t produce a return value.
- Return Mechanism:
Functions: Functions use a return statement to send a result back to the caller. The returned value can be stored in a variable or used in further computations.
Procedures: Procedures either don’t have a return statement or do not use it to return a result. While a procedure may perform a series of actions, it simply completes the task and hands control back to the caller.
Key Differences:
- Functions return a value after performing their task, allowing the caller to use the result in further calculations or processes.
- Procedures execute a task but do not return a value. Instead, they focus on performing actions or modifying the program state.
This distinction makes procedures useful for performing operations that don’t require feedback to the caller, such as printing messages, modifying variables, or triggering side effects, while functions are more appropriate when a result is needed for further use in the program.
Difference Between Function and Procedure: A Detailed Comparison
Both functions and procedures are essential concepts in programming, used to encapsulate blocks of code for reuse and better organization. However, they differ in several key aspects, including their purpose, syntax and structure, and execution behavior.
1. Purpose and Usage
The purpose and Usage of Function and Procedure are explained:
Function
- Purpose of Function: The primary purpose of a function is to perform a specific task or computation and return a value to the caller. Functions are typically used when the result of the operation is required by the program.
- Usage of Function: Functions are used when a program needs to compute or return a result, such as performing mathematical operations, transforming data, or processing user input. The returned value can be further utilized in the program (e.g., in calculations, assignments, or other functions).
Procedure
- Purpose: A procedure is used to perform a set of actions or operations, often modifying the program’s state, without returning any value. Procedures are used when you need to carry out a task (e.g., updating data, printing results) that does not require the return of a result.
- Usage: Procedures are ideal for tasks such as logging information, changing global variables, or interacting with external systems like databases or APIs. These tasks involve actions that affect the program’s state rather than producing a value for use elsewhere.
3. Syntax and Structure
- Function Structure: A function in most programming languages is defined by a return type, a name, and parameters (if applicable). The function contains a return statement that specifies the value being returned.
- Procedure Structure: A procedure is defined without specifying a return type. It may have a name, parameters, and an optional body, but it does not have a return statement (or its return statement doesn’t provide a value).
4. Execution Behavior
- Function Execution Flow: When a function is called, the program executes the function’s logic, computes the result, and then returns the result to the calling context. Once the function completes, it passes control back to the caller, and the value can be used for further operations.
- Procedure Execution Flow: When a procedure is called, the program executes the sequence of instructions within the procedure but does not produce a value to be returned to the caller. Once the procedure finishes, control is passed back to the caller.
Key Differences at a Glance:
Features | Function | Procedure |
---|---|---|
Return Value | Always returns a value | Does not return a value |
Purpose | Computes a result and returns it | Performs an action or operation |
Syntax | Includes a return type and return statement | No return type or return statement needed |
Use Case | Used for calculations or returning results | Used for tasks like printing, modifying data, or performing side effects |
Execution Flow | Executes, computes result, returns it | Executes instructions without returning a result effects |
Examples | Calculating a sum, transforming data | Logging an error, printing a message, updating a record |
Understanding when to use a function or a procedure is crucial for writing clear, modular, and maintainable code. Functions help in organizing code that produces results, while procedures handle actions that modify program behavior without the need for a return value.
Frequently Asked Questions (FAQs)
1. What is the main difference between a function and a procedure?
The main difference is that a function returns a value after performing a task, while a procedure does not return any value. Functions are used to compute results, whereas procedures are used to perform actions or operations.
2. Can a procedure return a value?
No, a procedure is designed to perform tasks without returning a value. However, some languages allow procedures to modify external variables, but they will not return a result to the caller.
3. When should I use a function instead of a procedure?
You should use a function when you need to compute a value that will be used later in the program. If you need to return a result after performing a calculation or transformation, a function is the appropriate choice.
4. Can a function perform actions without returning a value?
Yes, a function can perform actions (like printing to the screen or modifying variables) while returning a value. However, if the primary purpose is to perform actions without returning a result, a procedure may be a better choice.
5. Can a procedure accept parameters?
Yes, procedures can accept parameters, just like functions. These parameters allow the procedure to perform operations based on the values passed to it, but it will not return a result.
6. Is it possible to use a function for side effects?
While functions are typically used to return values, they can also have side effects (such as modifying variables or printing output). However, if the primary goal is to perform side effects without returning a value, a procedure is generally a better choice.
7. Do procedures require a return statement?
No, procedures do not require a return statement. They perform actions or operations and then return control to the caller without passing any result back.
8. Can procedures modify global variables?
Yes, procedures can modify global variables or program states, especially if they are passed as parameters or are part of the external environment. The key point is that they do not return a value.
9. Can a function be used to modify the program state?
While functions are primarily used to return a value, they can modify program state (e.g., changing a variable’s value). However, if the focus is on changing state without returning a value, a procedure may be more appropriate.
10. Are functions and procedures interchangeable?
While functions and procedures serve different purposes, they can sometimes be interchangeable depending on the situation. However, using a function when a return value is needed and a procedure when only actions are required helps maintain clarity and correctness in the code.
Conclusion
In conclusion, functions and procedures are both integral components of programming that help in organizing code and making it more modular, reusable, and efficient. However, they serve different purposes and are used in different scenarios:
- A function is designed to return a value after performing a specific task. It is used when the program needs to calculate or compute a result that will be used later in the code. Functions are primarily focused on producing a result based on input and are commonly used for calculations, transformations, and data processing.
- A procedure, on the other hand, is used to perform an action or execute a set of instructions without returning any value. Procedures are perfect for tasks such as modifying variables, printing outputs, interacting with external systems, or handling side effects. They focus on actions rather than producing results.
Understanding when to use a function or a procedure is crucial in programming. Functions are ideal for cases where a result is needed, while procedures excel at performing tasks without requiring a return value. By knowing their differences, you can structure your programs more effectively, improve readability, and create more efficient and modular code.
Ultimately, choosing between functions and procedures depends on the specific task at hand: whether you need to return a value for further use, or simply perform an action that modifies the state of the program.